Search algorithms are fundamental tools in computer science that enable efficient data retrieval. Whether you’re navigating through a vast database or searching for a specific item in a list, understanding these algorithms is crucial. This article, “Demystifying Search Algorithms: A Comprehensive Guide for Beginners in Computer Science,” aims to clarify the concepts behind search algorithms. We’ll explore the basics of linear and binary search methods, delve into their operational mechanisms, compare their performance, and discuss their real-world applications. By breaking down the complexities of search algorithms, we hope to equip beginners with the knowledge needed to effectively implement and optimize these essential techniques.
Join uzocn.com for a detailed examination of this topic.
1. Introduction to Search Algorithms
Search algorithms are a cornerstone of computer science, essential for efficiently locating information within data structures. At their core, these algorithms help us find specific elements in large datasets, making them vital for tasks ranging from simple list lookups to complex database queries. The efficiency of a search algorithm determines how quickly and effectively it can retrieve the desired data, which is crucial for performance in real-world applications.
In this article, we will focus on two fundamental types of search algorithms: linear search and binary search. Linear search is the most straightforward method, examining each element in a list sequentially until the target is found. Binary search, on the other hand, is more advanced and operates on sorted datasets, repeatedly dividing the search interval in half to locate the target more efficiently.
Understanding these basic search algorithms provides a solid foundation for grasping more complex techniques and optimizing data retrieval processes. By demystifying how these algorithms work, we aim to empower beginners wit
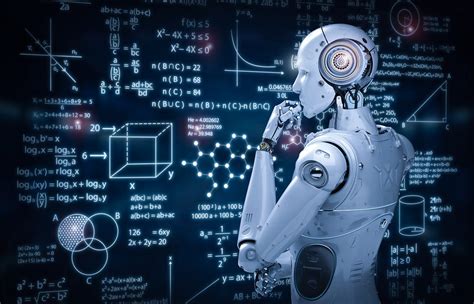
2. Types of Search Algorithms: Linear and Binary Search
Search algorithms fall into two primary categories: linear search and binary search.
Linear search is the most basic search algorithm. It operates by going through each item in a list one after another, starting from the beginning, until the desired item is found or the end of the list is reached. This approach is simple to understand but can be slow for large collections of data, as it might need to look at every single item.
Binary search, on the other hand, boasts greater efficiency but necessitates pre-sorting of the data. Its operation involves repeatedly halving the search interval. The process starts by comparing the target element with the middle element of the dataset. If the target is smaller, the search continues within the lower half; if larger, it continues within the upper half. This systematic narrowing of the search space significantly accelerates the process, making binary search markedly faster than linear search for extensive datasets.
Understanding these two fundamental search methods is essential for selecting
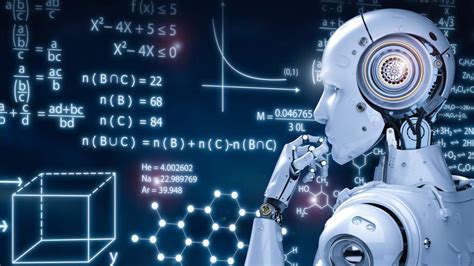
3. How Linear Search Works
Linear search is a simple algorithm for locating a specific element within a list. It works by systematically checking each element in the list, starting from the beginning, until either the desired element is found or the end of the list is reached.
Here’s how it works: the algorithm starts by examining the first element in the list and comparing it to the target value. If they are the same, the search is complete, and the position of the target is returned. Otherwise, the algorithm proceeds to the next element and repeats the comparison. This process continues until either the target is located or the end of the list is reached.
Although simple and easy to implement, linear search can be inefficient for large datasets, as it may require inspecting every element in the worst-case scenario. Despite this, it rema
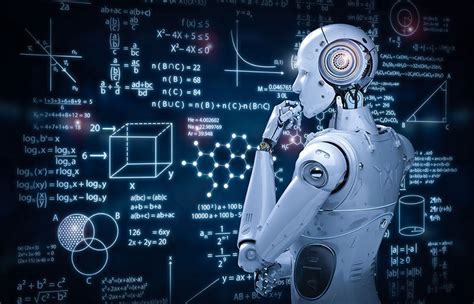
4. How Binary Search Works
Binary search is a more efficient algorithm designed for finding a target element in a sorted dataset. Unlike linear search, which examines elements sequentially, binary search takes advantage of the sorted order to reduce the number of comparisons needed.
The algorithm starts by comparing the target element to the middle element of the dataset. If the target matches the middle element, the search is complete, and the index of the target is returned. If the target is less than the middle element, the search continues in the lower half of the dataset. Conversely, if the target is greater than the middle element, the search continues in the upper half. This process effectively halves the search space with each comparison.
The binary search continues dividing the dataset and comparing until the target element is found or the search interval is reduced to zero, indicating that the target is not present. This method is much faster than linear search for large datasets, as it reduces the time complexity to O(log n), making it highly efficient for sorted data.
5. Comparison Between Linear and Binary Search
Linear and binary search algorithms differ significantly in their efficiency and suitability for various situations. Linear search, known for its simple implementation, exhibits a time complexity of O(n), indicating that its performance deteriorates proportionally to the size of the input. This makes it an ideal choice for small or unsorted datasets, where ease of implementation outweighs the need for speed.
Binary search, conversely, boasts a substantial performance advantage, exhibiting a time complexity of O(log n). This makes it significantly faster for large datasets. However, it necessitates prior sorting of the data, introducing an initial overhead. Once sorted, binary search efficiently narrows the search space, enabling rapid retrieval of the target element.
In summary, linear search is a versatile and easy-to-implement algorithm, but its efficiency decreases significantly for large datasets. Conversely, binary search, while highly efficient for sorted data, requires preprocessing to ensure the data is in order. Choosing the appropriate algorithm depends on the specific needs of the application, considering factors like data size, whether the data is already sorted, and the acceptable search time.
6. Real-world Applications of Search Algorithms
Linear search is a versatile algorithm with numerous applications in real-world scenarios. It proves particularly useful when dealing with small or unsorted datasets, making it ideal for simple database queries, small-scale data processing tasks, or situations where data is dynamically generated and not pre-sorted. Its straightforward implementation and simplicity make it an efficient choice for quick and uncomplicated searches.
Binary search is a vital algorithm used in various performance-critical applications that involve sorted data. For instance, it plays a key role in binary search trees, facilitating efficient data retrieval within computer science. Furthermore, databases and file systems utilize binary search to locate records or files swiftly. Crucially, search engines and indexing systems rely on binary search algorithms to handle massive amounts of sorted data, enabling rapid lookups and enhancing their efficiency.
Both search methods are essential components of everyday technologies, ranging from e-commerce platforms and search engines to software applications and data analysis tools. Their integration allows for efficient and effective data retrieval and management.
7. Time Complexity of Search Algorithms
Time complexity is a crucial factor in evaluating the efficiency of search algorithms. Linear search has a time complexity of O(n), where “n” represents the number of elements in the dataset. This means that in the worst case, the algorithm may need to examine every element in the list to find the target or determine that it is not present. As the dataset grows larger, the time required for linear search increases proportionally.
Binary search, however, operates with a time complexity of O(log n). This logarithmic time complexity indicates that the number of comparisons needed grows much slower than the size of the dataset. Binary search quickly narrows down the search space by repeatedly halving it, making it highly efficient for large, sorted datasets.
In summary, while linear search is simple and effective for smaller or unsorted datasets, binary search offers significant performance advantages for large, sorted datasets by reducing the number of necessary comparisons.
8. Space Complexity in Search Algorithms
Space complexity measures the amount of memory required by a search algorithm relative to the size of the dataset. Linear search has a space complexity of O(1), indicating constant space usage. This means it requires only a fixed amount of extra memory regardless of the dataset size. The algorithm operates in-place, scanning through the list without needing additional storage beyond a few variables for tracking the search process.
Binary search also has a space complexity of O(1) when implemented iteratively, as it uses a constant amount of extra space for variables like indices and the middle element. However, if binary search is implemented recursively, the space complexity can increase to O(log n) due to the call stack used for recursive function calls. This additional space is required to maintain the function calls and their associated data during execution.
In summary, both linear and iterative binary search are efficient in terms of space, though recursive binary search can require additional memory due to its stack usage.
9. Optimizing Search Algorithms
Optimizing search algorithms is essential for enhancing efficiency and performance, particularly when working with large datasets. While linear search, due to its inherent sequential nature, offers limited optimization possibilities, strategies like preprocessing can be employed in certain situations. Preprocessing involves techniques such as sorting data or utilizing more sophisticated data structures, effectively reducing the search space.
Binary search, inherently more efficient than other methods, can be further optimized. Maintaining sorted data and utilizing efficient structures like balanced binary search trees enhance its performance. Implementations can be refined to minimize comparisons or address specific data characteristics. Adaptive algorithms, for example, dynamically adjust their approach based on data distribution.
Furthermore, in practical settings, combining search algorithms with indexing methods can dramatically improve performance. Indexes, such as hash tables or B-trees, allow for swift data retrieval, reducing the necessity for exhaustive searches.
To summarize, although linear search offers limited opportunities for optimization, binary search can be greatly improved through strategic data organization, pre-processing, and efficient indexing techniques. These enhancements result in faster and more effective data retrieval.
10. Common Challenges and Solutions in Implementing Search Algorithms
Implementing search algorithms often presents challenges that require specific solutions to optimize both performance and accuracy. One common challenge with linear search is its inefficiency when handling large datasets, as it necessitates examining each element sequentially. This inefficiency can be addressed by utilizing more efficient algorithms such as binary search, although this approach requires the data to be sorted beforehand.
For binary search, a major challenge is ensuring that the dataset remains sorted, which can be resource-intensive. To address this, regular maintenance and efficient sorting algorithms should be used to keep the data in the required order. Additionally, binary search can face issues with recursive implementations leading to high memory usage due to the call stack. This can be mitigated by using iterative implementations, which have constant space complexity.
Another challenge is dealing with large-scale or dynamic datasets where frequent updates occur. In such cases, employing advanced data structures like B-trees or hash tables can provide faster search capabilities and handle dynamic changes efficiently.
Overall, optimizing search algorithms involves addressing these challenges through effective data management, choosing the
In conclusion, understanding and implementing search algorithms are fundamental skills in computer science. Linear and binary searches each have their strengths and limitations, making them suitable for different scenarios. Linear search is simple and effective for small or unsorted datasets, while binary search excels with sorted data, offering faster performance. By grasping their time and space complexities, as well as optimizing techniques and addressing common challenges, you can enhance your data retrieval strategies. Mastery of these algorithms lays a solid foundation for tackling more complex computational problems and improving overall efficiency.
uzocn.com